File I/O in Python – Read and Write Files Tutorial
The simple Python programs we write do not use persistent memory. Variables and objects we create in such programs are stored in the volatile memory (RAM). The data is erased when the program is closed. Most of the time, the program needs the data for later use. It must be somehow stored somewhere in the memory. In this tutorial, we will use Files to Read & Write data in Python. Once the data is saved, it can be retrieved and used later.
Basic Terminology related to File I/O
File
A File can be defined as a set of bytes written on the persistent memory, say hard drive. A has two important attributes:
- A file has a name
- A file has an extension
Writing data to File
The process of saving data in a File is called writing data to the file. When some data from a program is written to a file, then it is copied from RAM (Random Access Memory) to the file which resides in the hard disk. And such a File is called an output file because of the program stores output on it.
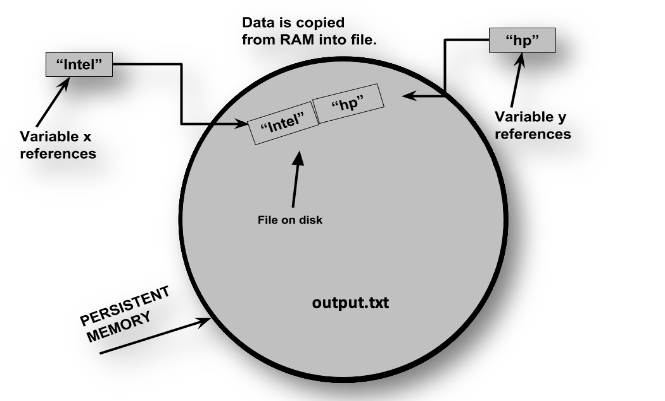
In the figure above, we have a file saved on a hard drive named output.txt
. Here, the output
is the name of the file and .txt
is the extension of the file. We have a variable named x
and a variable named y
that references to the strings “Intel” and “hp” respectively. These variables reside inside RAM. They are written on persistent memory as you can see in the figure.
Reading data from File
The process of retrieving data from the file is called reading data from the file. When some data is read from a File, it is copied from the file stored on persistent memory to RAM. Such a file is called the input file because the program gets the input from it.
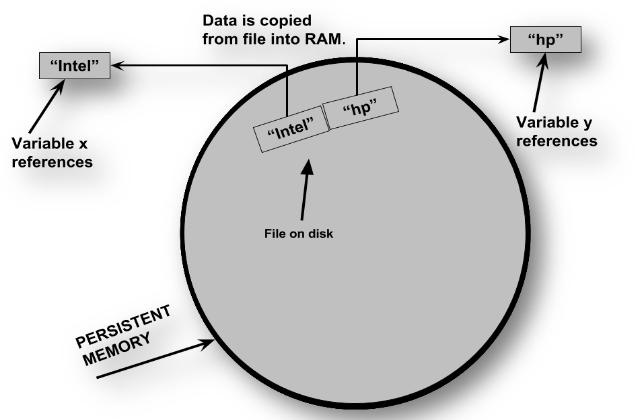
As you can see in the above picture, data is copied from File to RAM and stored in variables named x
and y
.
Common Way to Write Something
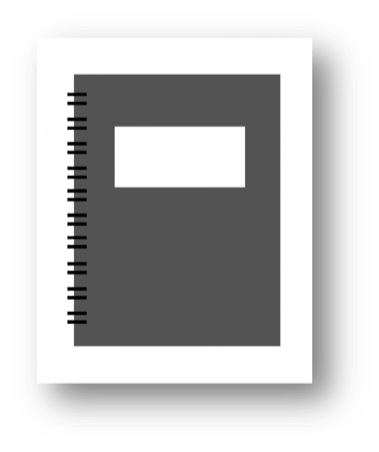
In our daily life we use writing pads, registers etc. to note down things. What we do is as follows:
- Open a file
- Process the file (WRITE / READ)
- Close the file
In a similar manner, we process files in programming. Let’s get started.
There are always three steps when we want the program to use a File for input/output.
- Open the file: In order to create a connection between a file and the program we open the file. Opening an output file usually creates a file on the disk if it already doesn’t exist and allows the program the write data to it. Opening an input file allows the program to read data from it.
- Process the file: At this stage, the program either write to the file or read from the file or can do both depending upon the nature of the program.
- Close the file: A file must be closed when a program finished processing it. When we close a file, it gets disconnected from the program.
File Access Methods
There are mainly two ways to access data stored in a file programmatically.
1. Sequential Access
In sequential access, you access the File data from the beginning of the File to the end of the File. If you need a piece of data that is stored near the end of the file then you have to go through all the data before it i.e. you have to read all the data and you cannot jump directly to the desired data. It is just like a cassette tape player.
2. Direct Access
Direct access file is also known as random access file. You can directly jump to the desired data without reading the data that comes before it.
In this article, we will use a sequential access file. Sequential access files are easy to handle.
File Object
In order to work with a file in a Python program, you must create a file object. A file object is an object associated with a specific file to work with programmatically.
That’s enough jargon. Let’s start coding.
Opening a File
Python provides open()
function to open a fileopen()
creates a file object and associates it with a file on your hard drive. The general syntax is:
fileVariable = open(filename, mode)
fileVariable is the name of the variable that references the file object.
filename is a string specifying the name of the file.
mode is a string specifying the mode (read, write etc.) in which file will be opened.
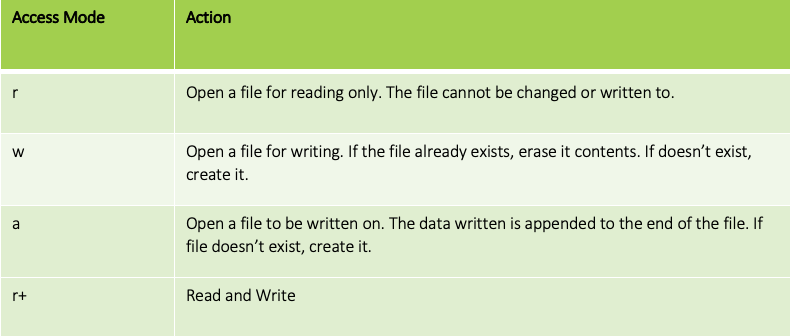
Suppose that we have a file named customers.txt
. To open this file following statement is used:
file = open(“customers.txt”) – default mode is read mode
file = open(“customers.txt”, ‘w’) – file opened in write mode
file = open(“customers.txt”, ‘a’) – file opened in append mode
file = open(“customers.txt”, “r+”) – file opened in read-write mode
Once a file is opened then we can read/write/append data to it.
Writing Data to File
In order to write the data to a File open the file in write or append mode. To write the data, you will use the file object and its methods.write()
method is used to write data to a file. A file must be closed after performing operations. close()
method is used to close the file.
The general syntax is:
fileVariable.write(string)
Example
Suppose we have a file named customers.txt
myFile = open("customers.txt", 'w') myFile.write("Customer 1 Qaiser 555\n") myFile.write("Customer 2 Fakhar 556\n") myFile.close() # this will close the file
The above-given piece of code will write a single line on file. You can write as many time as you want.
After the above-given piece of code runs, the customers.txt file will contain
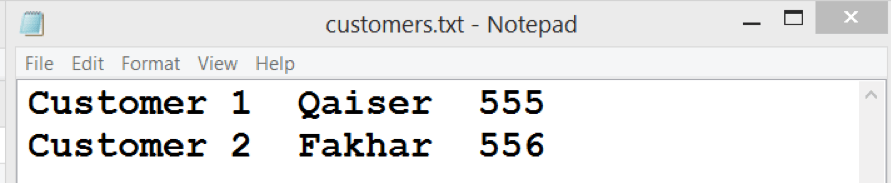
Writing Records to File
We can also write records to a File. For example, you have listed and you want it to be written on a File. It can be done easily. Create a file writing.py
.
#open a file with write mode file = open("brands.txt",'w') #We have some list and data of type string brands = ['Samsung', 'Apple','Nokia', 'Huawei', 'Oppo'] with file as f: for i in range(0, len(brands)): f.write(brands[i]+"\n")
Python provides a with
clause. It ensures that the file gets closed whenever the code inside the with
clause executes. When we use with
clause, we do not have to call the close()
method.
Writing Numeric Data to a file
Strings can be written directly to a File but the numbers must be converted into strings before they can be written. We can use Python’s built-in function str
that converts the value to a string.
Create a file called write_numeric_data.py
.
def main(): #Open a file for writing outputFile = open("numbers.txt","w") #Get three numbers from the user num1 = int(input("Enter first number:")) num2 = int(input("Enter second number:")) num3 = int(input("Enter third number:")) #Wrtie the numbers to file outputFile.write(str(num1) + "\n") outputFile.write(str(num2) + "\n") outputFile.write(str(num3) + "\n") #Close the file outputFile.close() print("Data written") #Call the function main()
Reading Data from File
In order to read the data from the File, we will use the read()
method. If the File is opened with ‘r’ access mode then we can read the whole content using read()
method.
read()
method returns the entire contents of a file as a string.
readLine()
method reads an entire line from a file. It returns the line as a string with newline escape character ‘\n’.
Reading All Contents of File
Create a file called read_all.py
. Suppose we have a file named users.txt
that contains some names.
def main(): #Open a file for reading #the access mode is r inputFile = open("users.txt","r") #read the contents from a file using read method data = inputFile.read() #print the data on console print("The Data of file is: \n") print(data) #close the file inputFile.close() #Call the function main()
Reading a whole line
Suppose we have a File with the following data

We can read this data line by line.
Create a file named readlines.py
.
#Open a file for reading #the access mode is r inputFile = open("data.txt","r") #read the line data = inputFile.readline() #print line data print(data) #read another line data = inputFile.readline() #print print(data) #close the file inputFile.close() #Call the function main()
Let’s write a program that reads numbers from a File and write the prime numbers on another File. We have a file named numbers.txt
that contains first 2000 consecutive numbers. We will write a naïve function to check whether the number is prime or not. If the number will be prime, we will write it on another file named primes.txt
.
Create a file fileio_example.py
#import math library import math as m #write a function called isPrime that accepts a number as #argument and returns true if the number is prime #otherwise returns false def isPrime(number): sqrt = int(m.sqrt(number)) for i in range(2,sqrt+1): if(number % i == 0): return False return True def main(): #Open file to read data infile = open("numbers.txt", "r") #output file to store primes outfile = open("primes.txt","w") #iterate through each line in file for line in infile: num = int(line) isprime = isPrime(num) if isprime: outfile.write(str(num) + '\n') infile.close() outfile.close() main()
Thank you for reading the Tutorial. I hope It will be helpful. Comment If you find any difficulty.
Here’re some more Articles, you might be interested:
— Python Sqlite3 Tutorial – Database Operations With Code Example
One Comment
Comments are closed.